Introduction
Imagine you are a service based company or a freelancer who constantly wants to make connection and increase your network for better opportunities or a job seeker who want to get more visibility among companies for better opportunities then the best way to get more opportunities is by sending cold emails to companies or organizations.
š”You can get all of the code shown in this blog from here.
What is cold email?
Cold email is a marketing strategy where a person or organization sends emails to potential clients or customers who havenāt expressed prior interest in their product or service. The main goal of Ā cold emailing is to initiate contact and generate interest of leads.
To get more replies and interest from your leads, you need to first research about the different domains and different leads in those domains. You canāt send a same email to everyone because if you are selling any service then you must target different domains differently to make more impact and your leads can relate to your service. It is also very important with additional emails to encourage them to take action after sending your first cold email.
Which workflow we are automating?
There are many services to manage your cold email workflow for example smartlead and instantly but these software does require some manual setup. Also you have to reply to every lead manually even if it is a small question which can be answered by any bot.
Even if you create a basic bot using any scripting language for this flow which can be triggered for every email message then still it wonāt be that much impactful for your leads because as we discussed above, we need to give personal touch to every conversation because in cold email, you are interacting with lead to get an opportunity and to make a good impression you have to handle every email carefully and thatās why you canāt have a basic bot to handle this job for you because it canāt talk in a way that you talk generally in your email conversations.
Now imagine you have written a very good email which increased the reply rate for you and now you are getting so many replies and as a good CEO or freelancer you want to answer every email to make the best impression š but it is taking too much time for you and you canāt focus on other things in company rather than replying to your emails. But what if i tell you that you can have a custom autonomous AI agent who can talk like you, reply to your emails and also classify your emails for you, sounds great right? š
In this blog, we are going to automate this exact email replying workflow for rohan who is CEO and founder of Ionio so we will create a autonomous AI agent completely from scratch which can reply to his emails in his tone and also classify his emails based on conversation so letās start š!
ā
Workflow of our agent
Letās take a look at workflow of our agent š !
The agent will take the campaign id and the csv file of lead emails whom you want to reply. Once that is provided, it will first classify the emails based on the conversation and if the email is positive or needs reply then it will construct a reply for that. Generally we donāt need to reply for negative emails or blank emails so this classification will help us to find the category of email.
We will require 4 tools for this workflow:
- Email Classifier Tool: It will be used to categorize emails based on the email conversation
- Company Search Tool: It will be used to get the information about any person or organization using apollo API
- Email Writer Tool: It will be used to construct a email reply for given message.
- Email Sender Tool: It will be used to send the email reply to given lead using smartlead API
This is how the flow will look like:
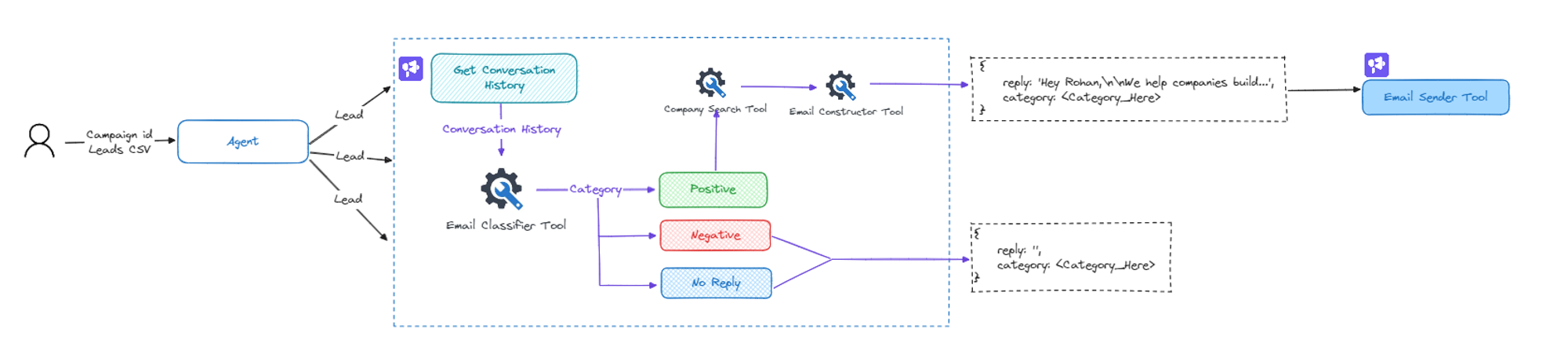
ā
Prerequisites
Now there are some prerequisites which we need to setup so letās take a look at them!
- First of all, we are going to use SMARTLEAD as our cold email management software so i encourage you to first create an account on smartlead and create one campaign. After creating a campaign, add your leads as a csv file and then you can follow the remaining steps provided on the SMARTLEAD dashboard to start your campaign.
- We will be using smartlead API to get the conversation history and also we will use it to reply back to lead. Get your API key from smartlead by going to settings ā Your profile ā smartlead API Key and copy your api key. If you are using any other cold email software, then read their API documentations and follow accordingly.
- We are going to use GPT-4 as our LLM model for agent so get your openai API key from openai dashboard.
- We are also going to use apollo API to get the information about lead and itās organization. we will talk about it more later in the blog
ā
Letās setup our knowledge base
Once you have successfully created a campaign on smartlead and you are getting replies from your leads then itās time to create our knowledge base because to make an agent reply in your tone, we need to feed your past emails and replies to it and then using vector embeddings we can filter out top matching results for current reply and generate our custom reply using LLM(Large language model).
Basically to use this agent, you will have to provide 2 CSVs to our agent:
- Past emails and replies between you and leads
- FAQs about you and your organization
We will get the email and reply CSV from smartlead and from that CSV, we will generate our FAQs.
Letās take a look at how you can generate these 2 CSVs!
Getting past emails and replies
You can get the past emails and replies from smartlead by going to your campaign ā inbox. In inbox, filter the leads who have replied to your email from sequence status filter.
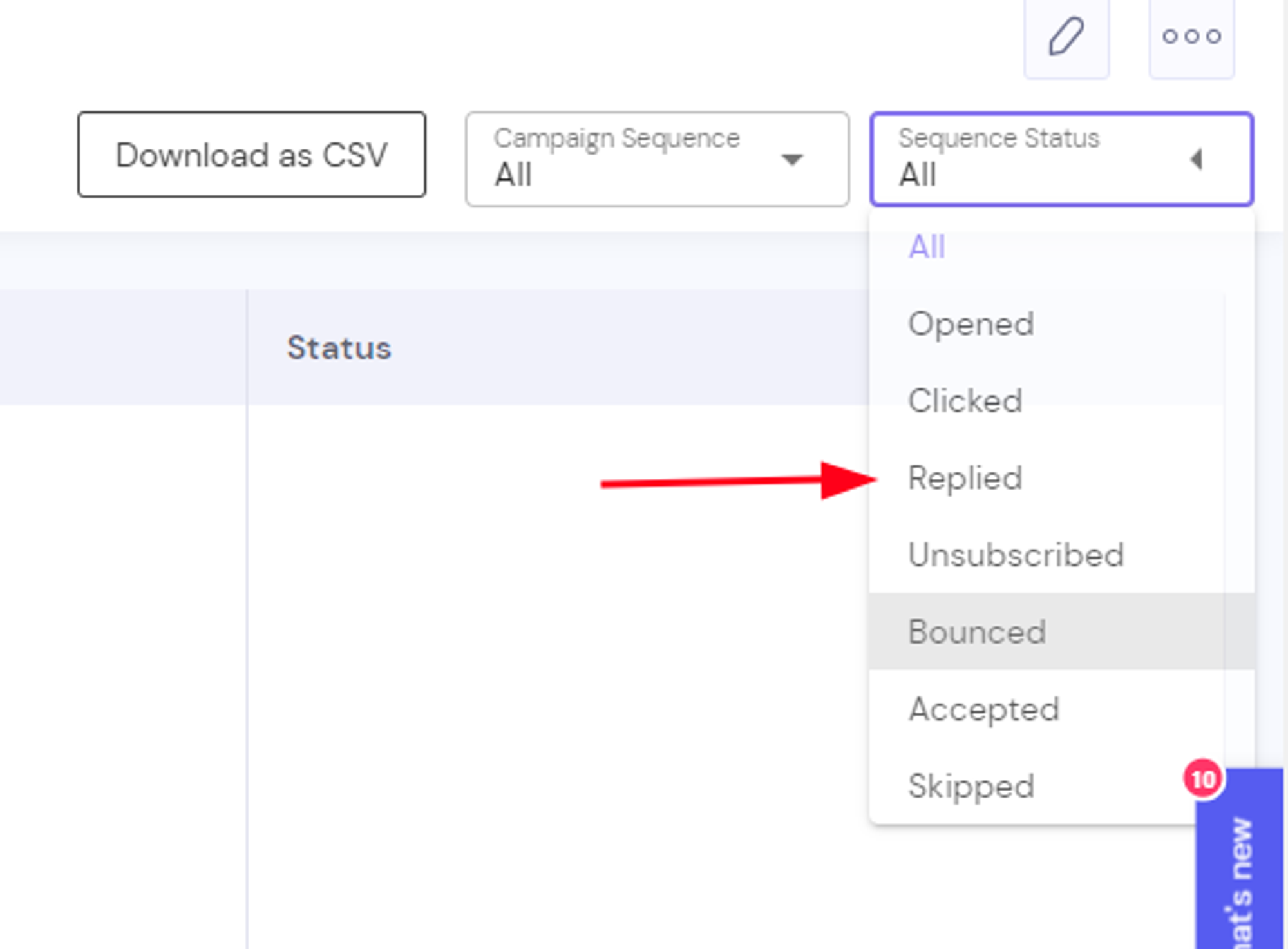
After that click on āDownload as CSVā button to get these leads.
Now we canāt directly create embeddings of this csv because it contains full email threads as a plain text and we have to format it to make it limited to single message and single reply so letās write some code for it š§āš»
First install the required dependencies
Load your api keys from environment variables
Import the required dependencies
To get the message history for any lead, you will need a campaign_id of the campaign for which you want to fetch the conversation. You can get the campaign_id from āList all Campaignsā endpoint of smartlead API:
From the given array of objects, you can get the campaign id of your campaign.
Once you got the campaign id, itās time to create our knowledge base.
We have all the lead information which we downloaded from smartlead in āLead_Data.csvā file and after formatting each reply, we will store it in a new csv file called āfinal.csvā and it will have only 2 columns called "Message and Reply"
Your final csv file will look like this:
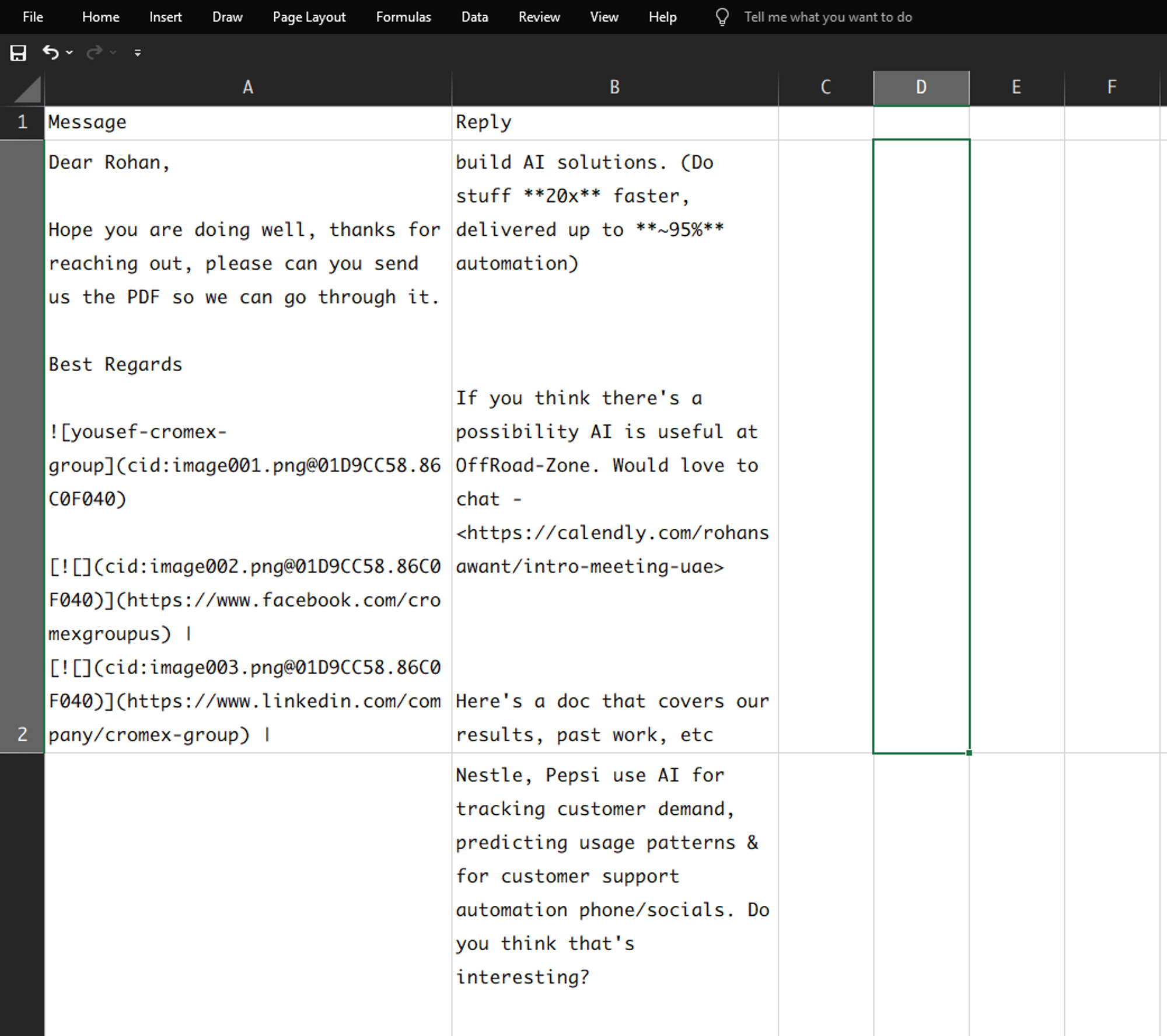
Creating FAQs
Now letās create our 2nd knowledge base which is FAQs about you and your organization
To create faqs, we will get all the replies from the āfinal.csvā file which we just created and generate some quetions and answers about you and your organization from those replies so that our agent can use this information as a reference. You can also use your own FAQs instead of generating them from emails.
Here we will use textsplitter from Langchain because we canāt pass this whole csv content at once in prompt so we will convert the csv content in chunks and generate FAQs for that specific chunk and at last we will combine every chunk faqs in single array of json.
Letās first install langchain module
Now letās write logic for extracting FAQs from our csv file
But there is one problem ā¹ļø, for some reason i was not getting the full array of json as a response as you can see in the below screenshot:
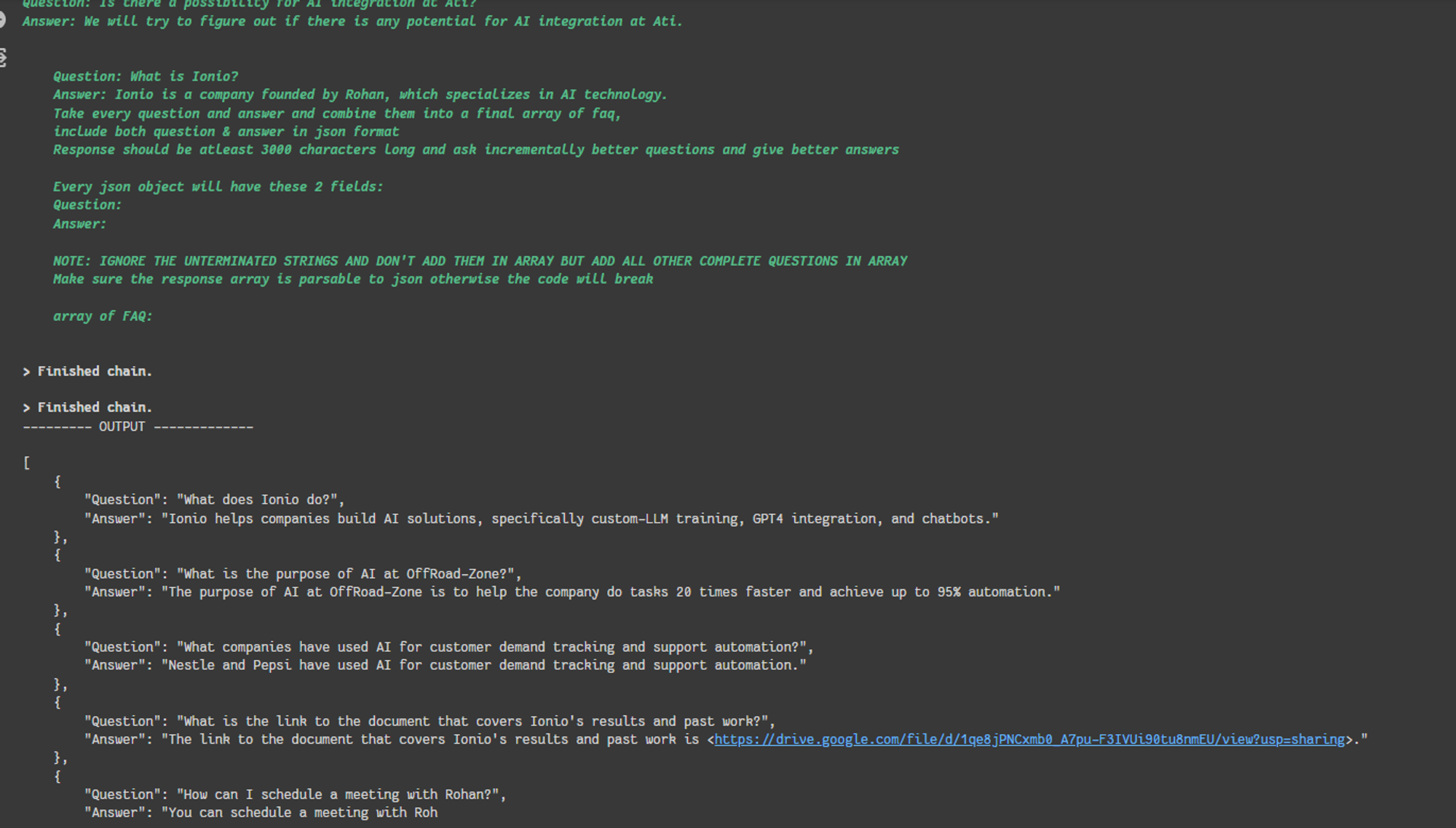
It could have happened because of the langchain system prompts or the context window problem and i tried to make it work with different chunk sizes and llms but it didnāt work. So instead of wasting time on this i just passed all the faqs from different chunks and passed them to chatgpt and it gave me json file of FAQs.
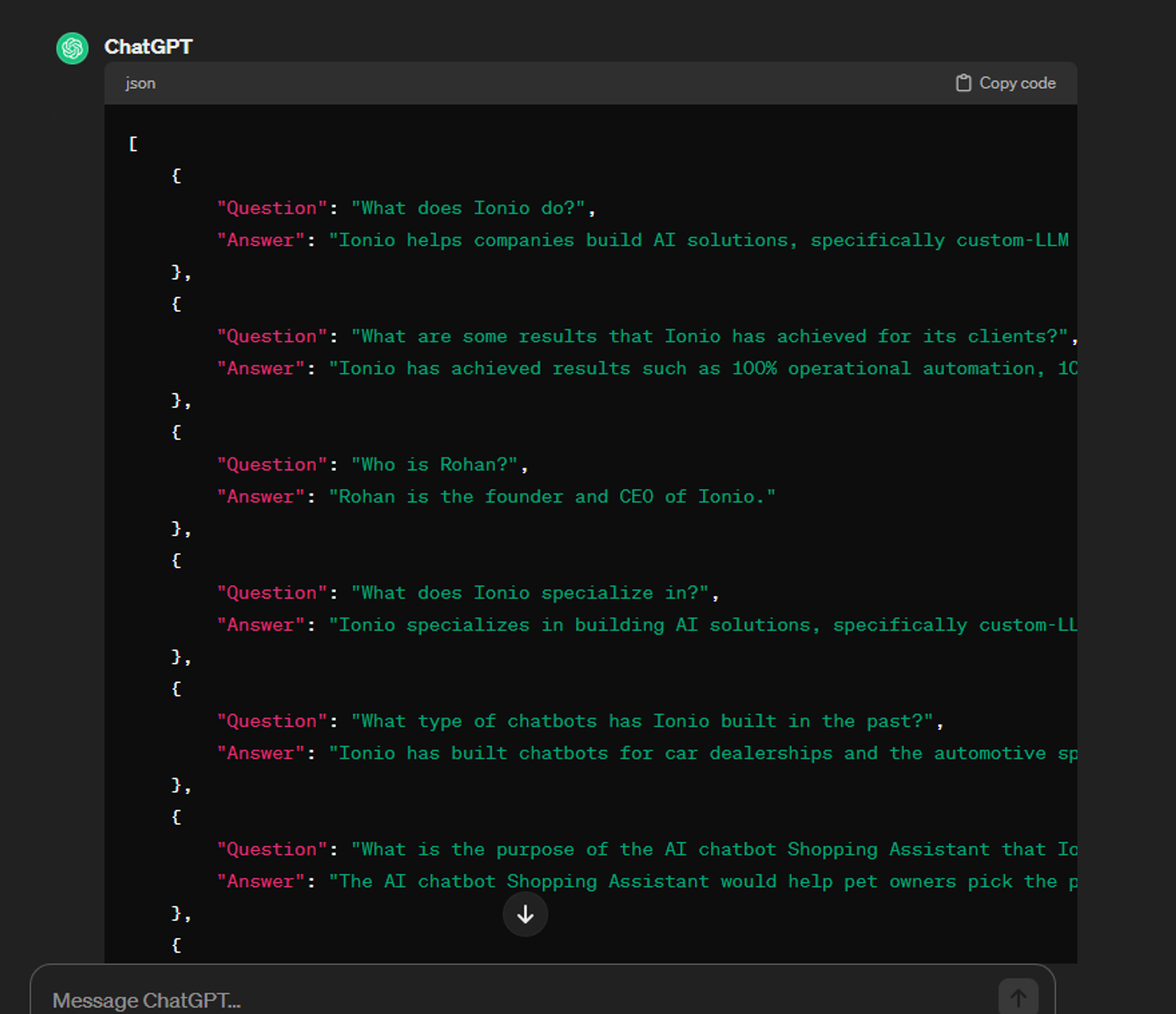
Take this json data and store it in file called āfaq.csvā
Now we have both CSVs ready, itās time to create our agent š¤
ā
Creating our agent
Now we have all the things ready which we want to create our agent so letās start creating our agent by importing required dependencies
Now letās create our custom tools!
Email Categorization Tool
Once we get the email conversation from smartlead, the first task of agent is to categorize this email based on the email conversation and then decide whether to reply to this email or not. We will use gpt-4 as our LLM model here to categorize these emails.
This tool will take only 1 input parameter:
- Conversation: Past email conversation between rohan and lead as an array of json objects where each object contains sender and message field
We will categorize these emails in total 8 categories but you can customize these categories according to your needs and use case. Letās take a look at prompt which we are going to pass to our LLM.
Letās write code for it!
Company Search Tool
To give more personal touch to every email, it is good to search about lead and itās organization first before booking a meet with them and if you mention it in your email reply then it can make a good impact which shows that the person sending an email is real person who searched about your organization and is interested to work with you š
To search about any organization, we will use apollo API which will give us information about any organization from the lead email.
This tool will take 2 parameters as input:
- Email: Email of lead
- Category: Category of email conversation (we are passing this because we want this tool to run only after categorization is done)
So letās create our tool!
Email Writer Tool
Now itās time to create the core tool of whole workflow because the main goal of this agent is to mimic the tone of rohan and reply in a way in which rohan replies to his cold emails. So we will have to provide all the required information and a detailed prompt to this tool so that we can get more better results.
The basic idea of achieving this will be like this:
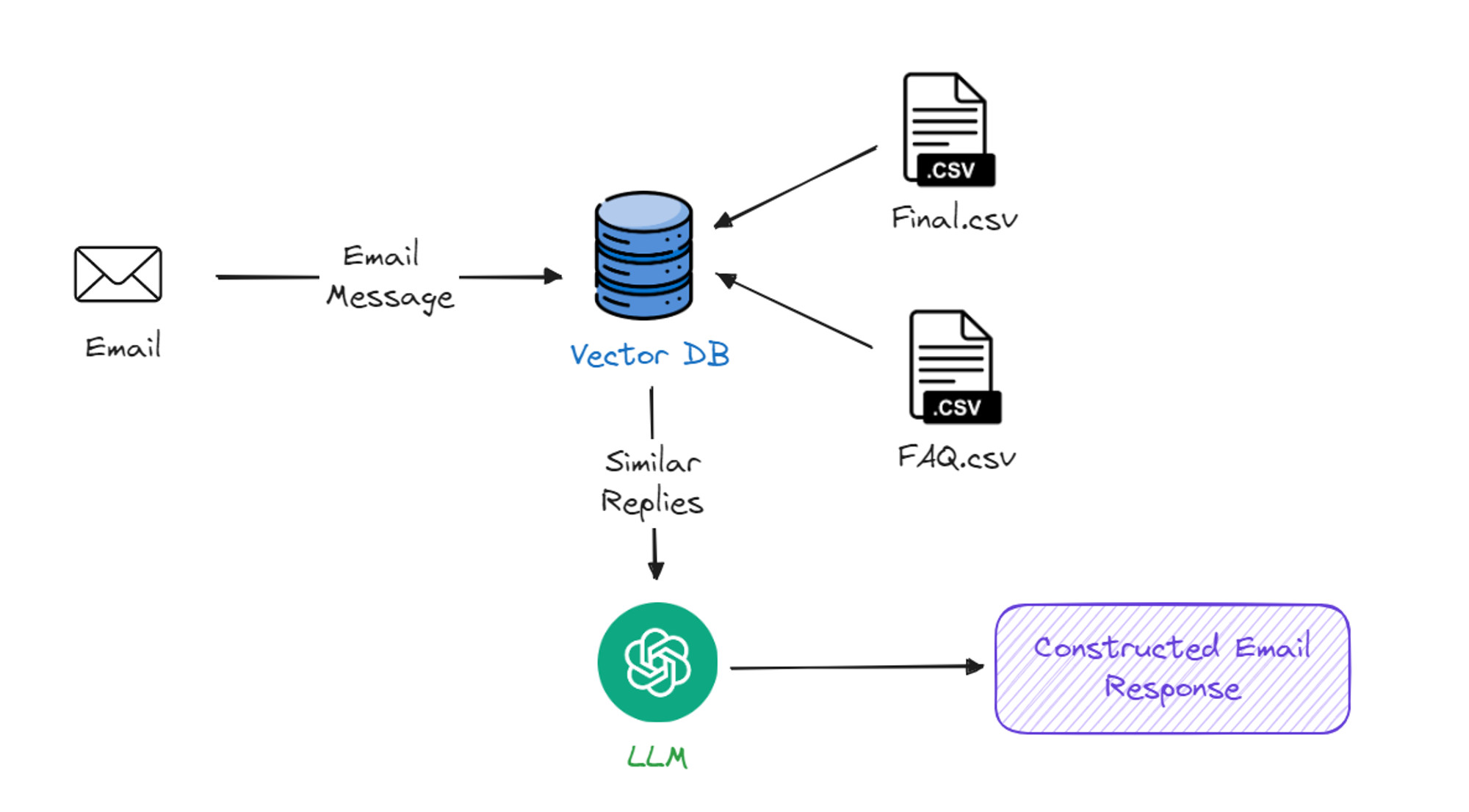
We can write a code for this entire architecture where first we have to store our CSV knowledge base in a vector database and then based on the user input we can perform semantic search on the message and FAQs. Once we got all the similar email and reply pairs, we can pass this to LLM and it will construct an email response for you which mimics the tone of rohan.
For this blog, i am going to use relevance platform where we can create our custom AI tools and host them. The main advantage of relevance is it comes with pre built vector database where you can store your data and then use that data in your tools and you donāt need to worry about any vector embedding or semantic search process.
First of all, goto relevance dashboard and add the āfinal.csvā which contains message and reply pairs for your past emails:
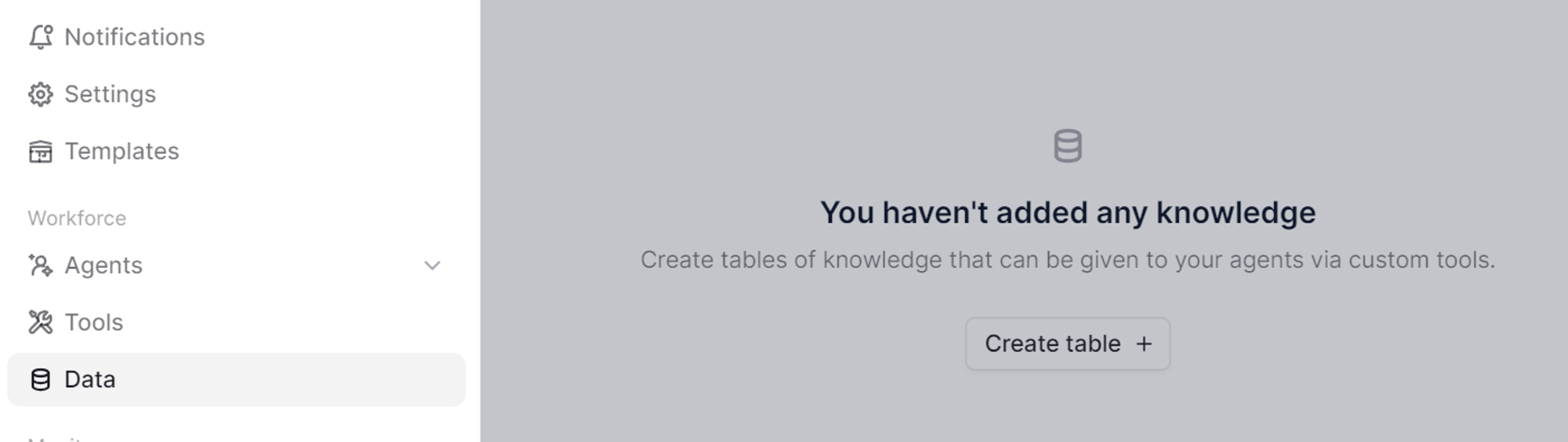
When it asks for āwhat content should be added to knowledge?ā then only select message because we only want to vectorize the messages and we are going to perform semantic search on these messages with given lead message for which we have to generate a reply
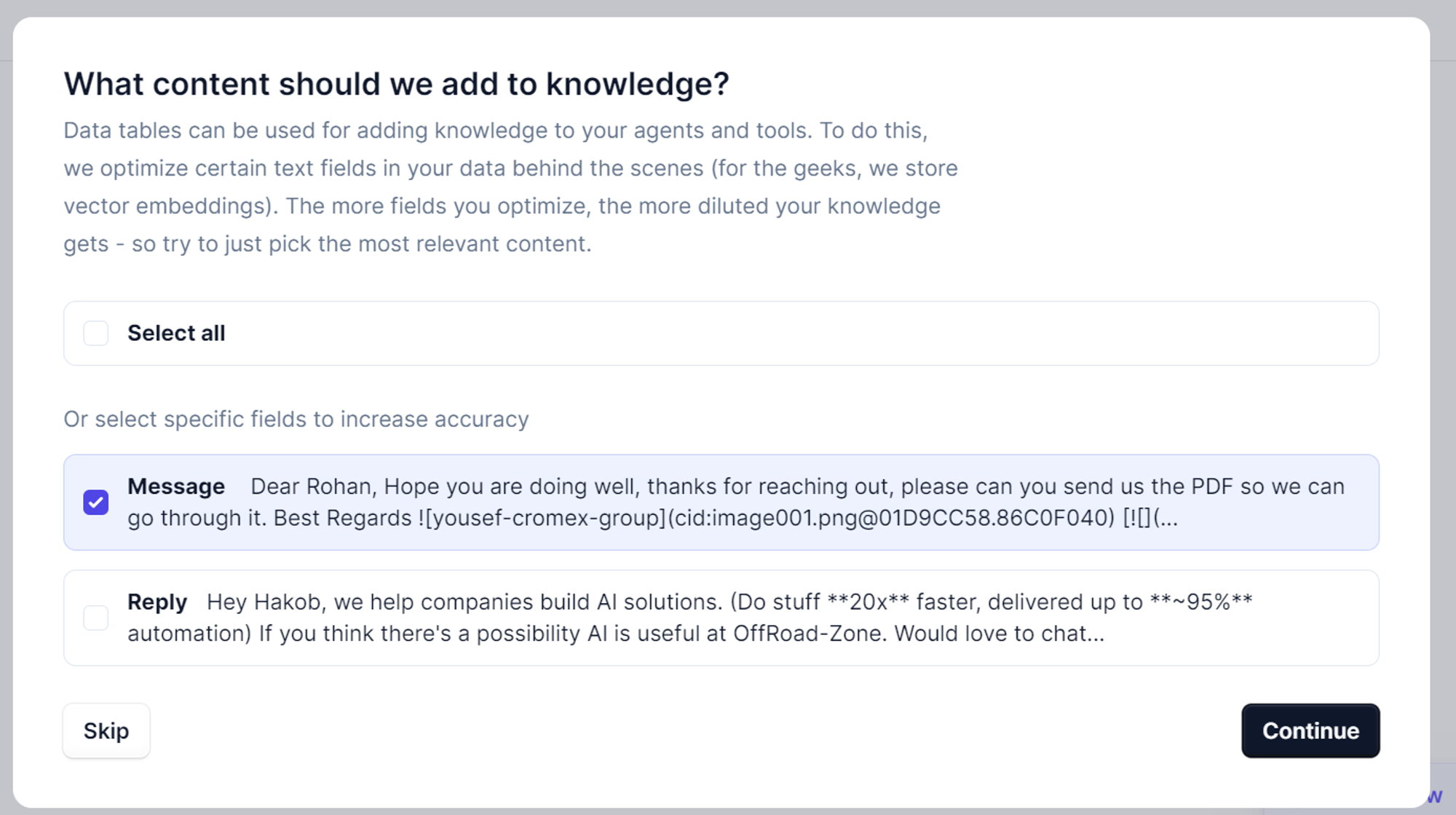
Once you have created a table for message and reply pairs, its time to add our āFAQ.csvā file in this knowledge base. This time we will vectorize both question and answer.
Now we have uploaded both CSVs, itās time to create our tool

Follow these steps to create your tool:
- Go to tool section from navbar and click on ācreate new toolā and select āstart from scratchā.
- Add your knowledge base in knowledge section.
- Specify your user inputs in input section. We are going to use 4 inputs here:
- Client Email: The email text for which we have to construct a email reply
- Sender Name: The name of sender
- Conversation History: Conversation history between you and sender
- Company Description: Description about leadās organization
- At last, select your LLM and add the below prompt in prompt section:
Now we are ready to use this tool š!
Fill the required input values and click on ārun stepā and we can see that i am getting the reply which looks exactly how rohan talks in his emails š!
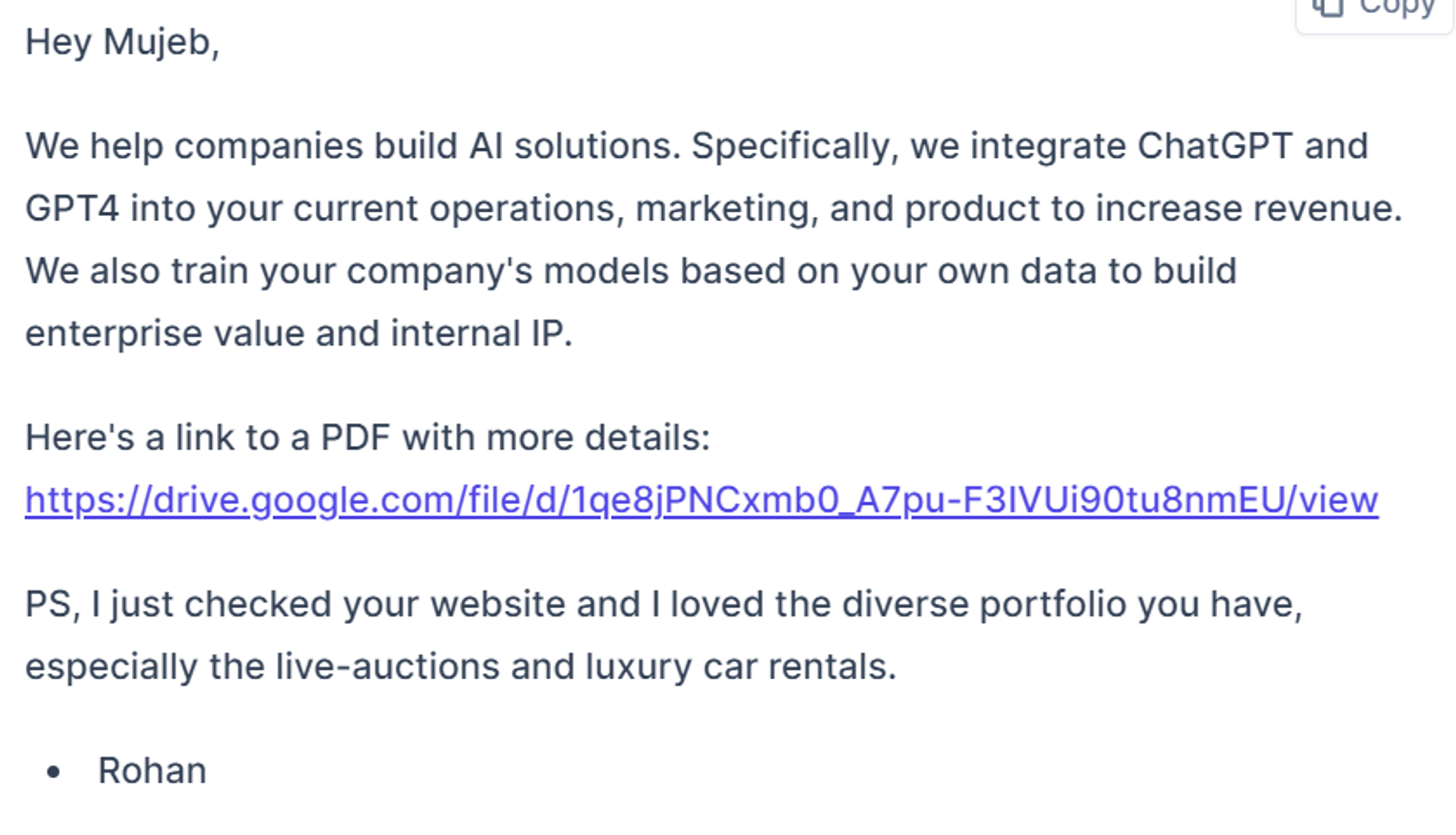
Now itās time to integrate this tool in our agent so click on āAPIā section and click on āDeployā and it will deploy this tool for you for free and you can trigger this tool by making the POST request to given API endpoint.
This email writer tool will take the same 4 parameters which we used as an input in the tool which we created on relevance because we are going to pass these 4 parameters as a input to that tool.
Here is the code for email writer tool:
Email Sender Tool
Letās create our last tool which is email sender tool (we can trigger this function with agent too but instead of passing so many parameters to agent, we can just call it after agent gives us reply) which will help us to send emails back to leads using smartlead API. we will require some parameters mentioned on their API documentation page to send an email.
Now letās initialize our agent by providing a system prompt, memory and list of tools to it!
Now we are ready to use this agent and we will take 2 things from user before running this agent:
- Campaign ID: Campaign id of the campaign for which you want to run this agent
- Lead CSV: The CSV file of leads whom you want to reply or categorize their replies (follow the same procedure which we discussed while building our email/reply knowledge base to get the csv file)
Once we get the CSV file of leads, we will traverse every lead conversation one by one and run agent for every lead and we will reply to them if needed.
After running the above code, you will see that agent is running for every lead one by one and replying to leads if needed.
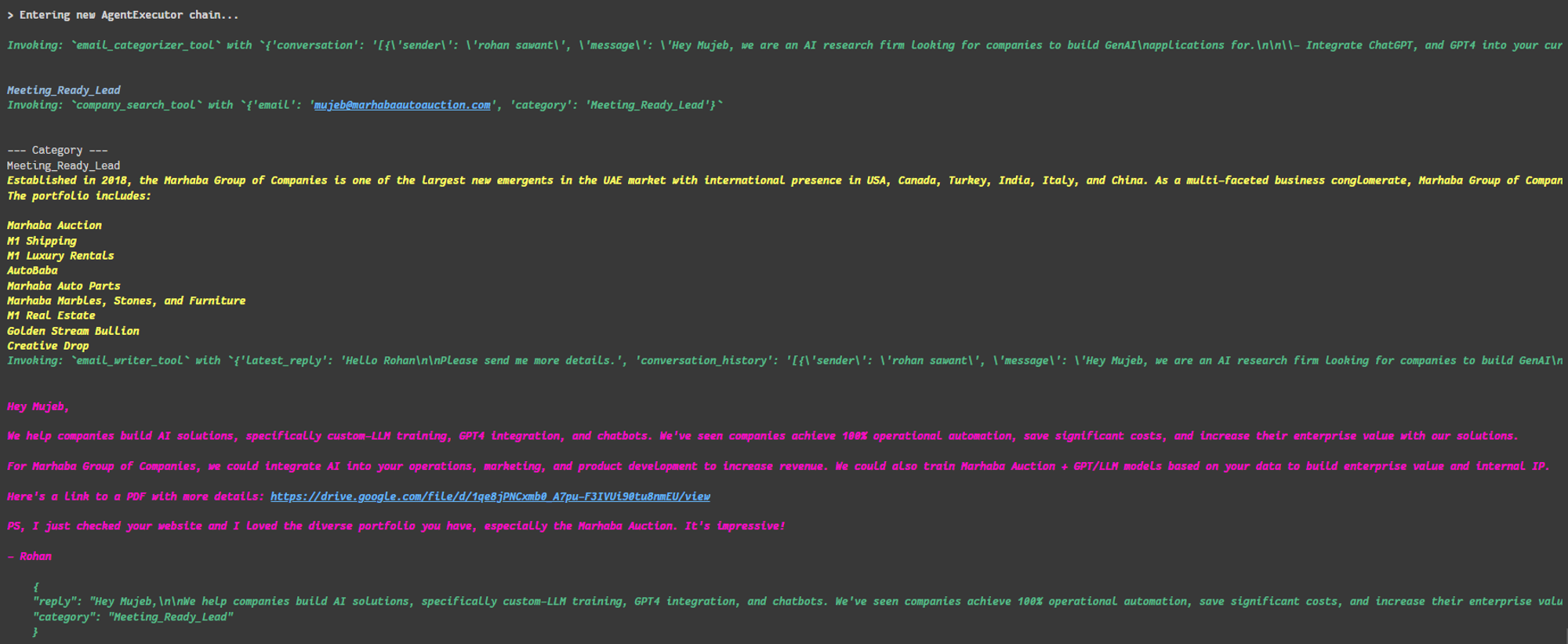
It is also able to categorize different type of emails

And we have successfully automated the cold email workflow using our agent š
š”You can get all of the code shown in this blog from here.
ā
Challenges
Letās discuss the challenges which i faced while making this agent š
Filtering Leads
The first issue i faced was related to filtering the leads because there are thousands of leads in every campaign and you canāt just check every lead one by one and check if they have replied to your email or not and then run the agent because it will take so many API requests and you might face rate limit error.
So instead of checking every lead, i decided to get only those leads who have replied to my first cold email and in smartlead you can easily get it using filters provided on the platform and you can easily export then in CSV file. If you are using any other cold email software then there must be similar option to filter out leads based on their reply status.
Once you got the leads who have replied to your email, you can then get the conversation history between you and lead and check the sender of last message and if it is lead then you need to reply to that message otherwise you have already replied to their message then you donāt need to run agent (If you want to send a follow up message then you can run that agent with some changes in prompt for followups).
Formatting The Email Data
The email conversation history which i was getting from smartlead API was not properly formatted because i was getting the email thread as html and even after converting that html into plain text it was looking like this:
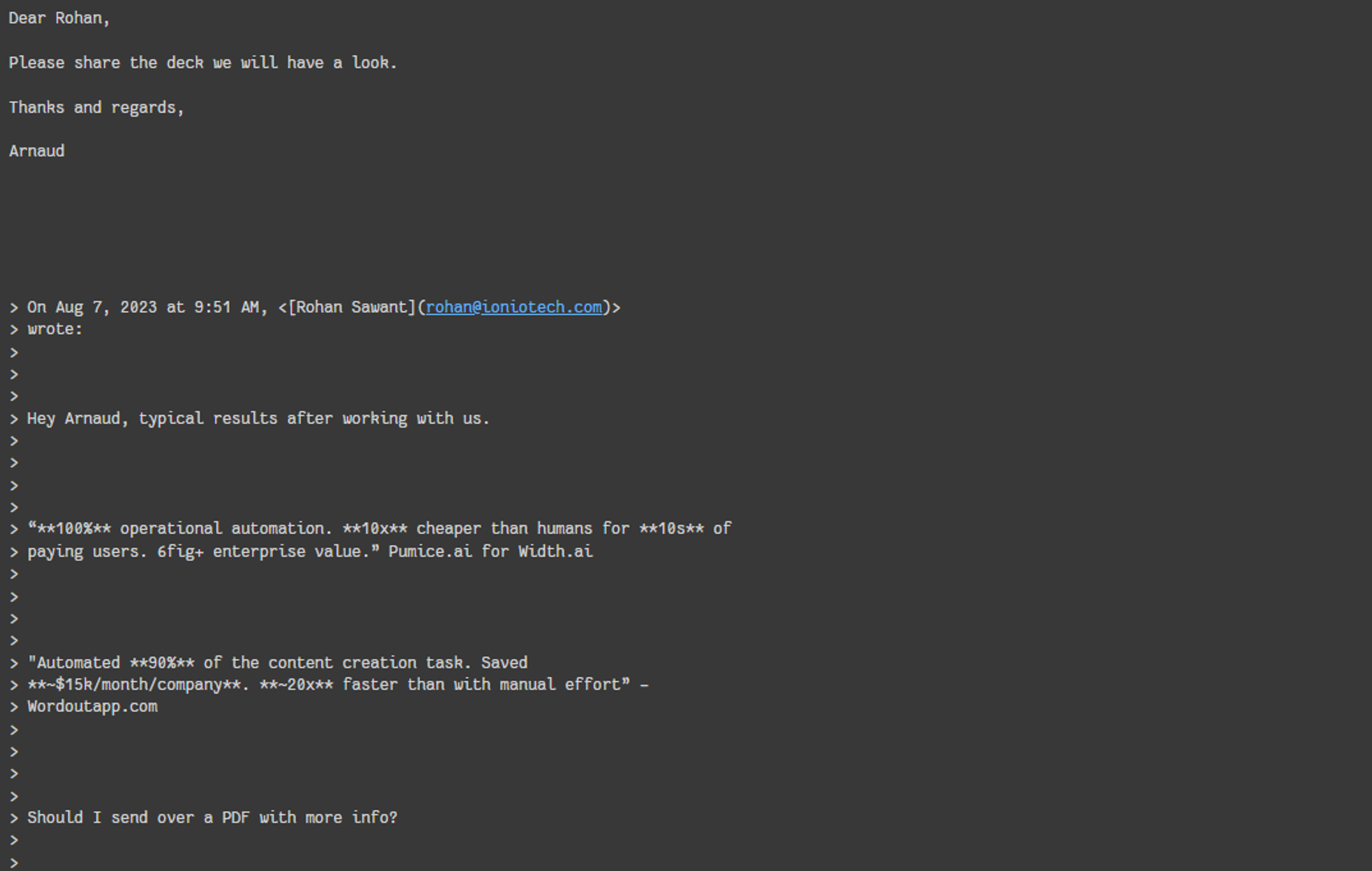
To get only the latest message from long email thread, I used openai to format it properly and then i was able to get the latest message from this long email thread without any extra space or information.
To build the conversation history array, I traversed the array coming from smartlead API and for every element in that array, I formatted it with openai and then added it into our conversation history array.
Generating Reply in Human Tone
The main aim of agent was to generate replies in any humanās tone and to achieve that i first tried with only the email/reply knowledge base and FAQs in prompt but still it was not perfect. There were still some issues like:
- It was repeating the question or content from leadās message ( For example, if lead says they are interested then agent was saying āI am glad that you are interestedā and it looks bot generated text)
- The message was not concise and descriptive but it was just looking like a long paragraph (Rohanās previous replies were short and concise so we need to match that pattern)
- Agent donāt have context of full conversation because it only have last message
To solve this issue, i added some conditions in LLM prompt which LLM should follow and then i got better results and also i then added conversation history as a input parameter to our email writer tool.
To give more human touch to our email response, I then decided to search about lead and their organization. So first i decided to scrape their website but not every website allows scraping so you might not get much information so i used apollo API to get information about lead and their organization and then on every email reply i added one extra line which tells them what i liked about their organization or website to make it look like more natural and human.
It was looking like this before adding above conditions:

This is how it looks after applying all the changes:

You can also search about leadās latest achievements or news from internet and add that too and also you donāt need to add it in response everytime so you can tell the agent when you need to add this line.
ā
Conclusion
The main goal was this agent to handle every lead reply very carefully because as i said before, the reply rate for some emails might not be high so you need to handle every lead very carefully and donāt make them feel like you are just spamming on every lead and you have made some sort of bot script which replies to your emails because then they might lose interest.
AI agents are next big thing in AI-ML field and many companies are using them to automate their workflows because if you hire a inbox manager or email writer for your companyās cold emails then it might cost you at least $50k a year. As we saw in blog, itās very easy to setup an autonomous agent for your organization using langchain and python knowledge.
Want to Automate Your Workflows?
So, whether you are a small team looking for clients, a job seeker looking for better opportunities, a freelancer looking for clients or a large organization seeking for more clients then cold emails are one of the best ways to get more connections and AI agents can automate this work for you.
If you are looking to build custom AI agents to automate your workflows then kindly book a call with us and we will be happy to convert your ideas into reality and make your life easy.
Thanks for reading š.